The “Two Sum” problem in LeetCode is a classic problem in computer science that asks you to find two numbers in an array that add up to a specific target. Here’s how you can solve this problem:
- Brute Force Solution: The simplest way to solve this problem is to use a brute force approach that involves checking every possible pair of numbers in the array. For each pair, you check if the sum of the two numbers equals the target. This approach has a time complexity of O(n^2), where n is the number of elements in the array.
Here’s an example code for the Brute Force solution:
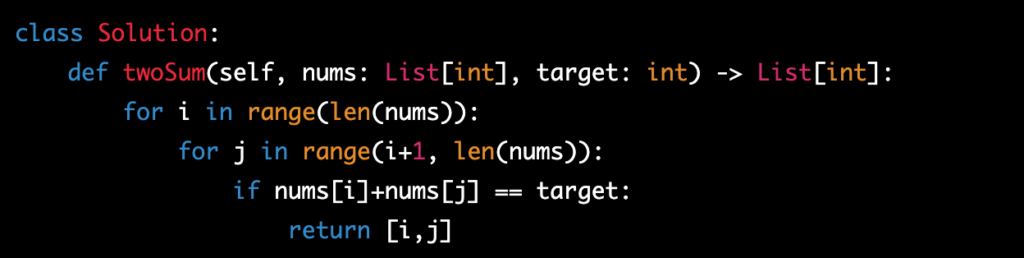
- Hash Map Solution: A more efficient way to solve this problem is to use a hash map. You can loop through the array and for each element, you can check if the difference between the target and the current element is already in the hash map. If it is, you have found your solution. If not, you add the current element and its index to the hash map. This approach has a time complexity of O(n), where n is the number of elements in the array.
Here’s an example code for the Hash Map solution:
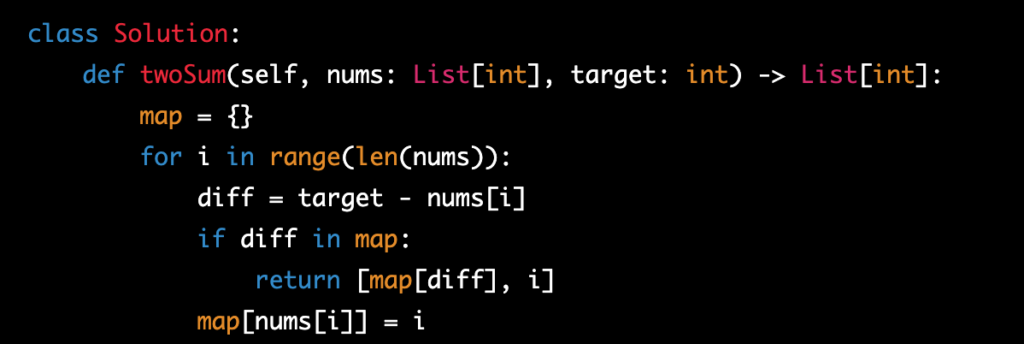
Both the Brute Force and Hash Map solutions have their own pros and cons. The Brute Force approach is simple to understand but has a higher time complexity. The Hash Map approach is more efficient but requires more memory to store the hash map.